Obj Surface Face DistributionΒΆ
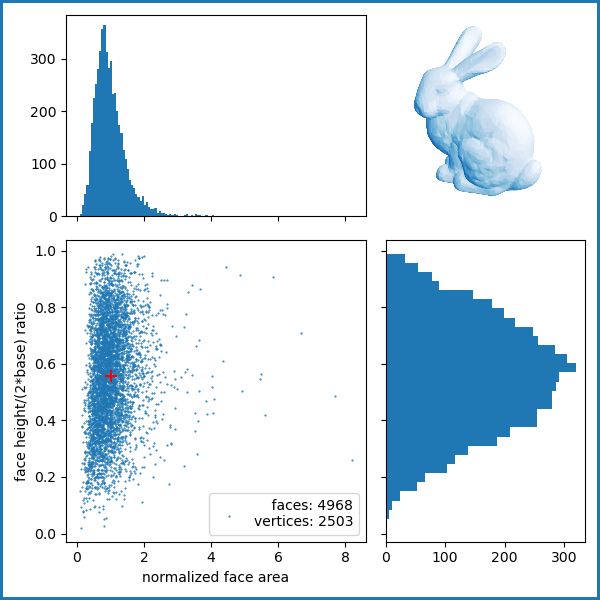
The surface property, area_h2b, is a 2 X N array of areas and height to base ratios of the N polygonal surface faces. Areas are normalized with respect to the average face area. The height to base ratios are normalized so that equilateral triangles and squares have a value of unity.
import matplotlib.pyplot as plt
import numpy as np
import s3dlib.surface as s3d
# Obj Surface Face Distributions
# Setup surface ................................................
objPath = "data/bunny.obj"
surface = s3d.get_surfgeom_from_obj( objPath )
surface.map_cmap_from_normals('Blues_r')
ah2b = surface.area_h2b
info = ' faces: '+ str(len(surface.facecenters.T)) + \
'\nvertices: '+ str(len(surface.vertices.T))
# Construct figure, add surface & plots ........................
fig, axs = plt.subplots(ncols=2, nrows=2, figsize=(6,6),
width_ratios=[.6,.4], height_ratios=[.4,.6],
layout="constrained",linewidth=3,edgecolor='C0')
ax = axs[1, 0] # ... scatter plot
ax.scatter(*ah2b,s=1,marker='.',label=info)
ax.scatter(*np.mean(ah2b,axis=1),s=80,marker='+',c='r')
ax.set_xlabel('normalized face area')
ax.set_ylabel('face height/(2*base) ratio')
ax.legend()
ax = axs[0, 0] # ... face area histogram
ax.xaxis.set_tick_params(labelbottom=False)
ax.hist(ah2b[0], bins='auto')
ax = axs[1, 1] # ... h2b ratio histogram
ax.yaxis.set_tick_params(labelleft=False)
ax.hist(ah2b[1], bins='auto', orientation='horizontal')
axs[0,1].set_axis_off() # ... hide 2D axis, add 3D axis
ax = fig.add_subplot(2,2,2, projection='3d', aspect='equal')
ax.set_axis_off()
s3d.auto_scale(ax,surface, uscale=0.8 )
ax.add_collection3d(surface)
fig.tight_layout()
plt.show()