Klien GlassΒΆ
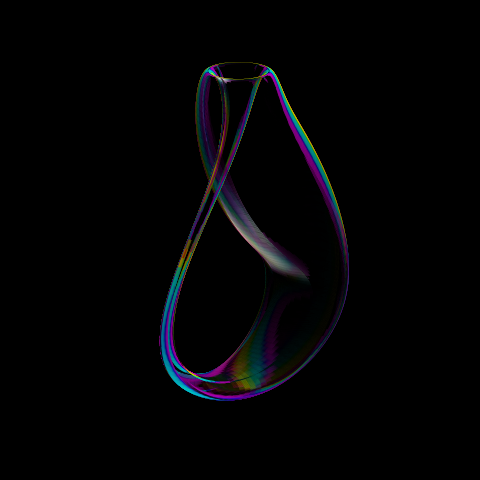
In this example, a binary colormap is composed from two colormaps which are mirrored from each other. Both colormaps are themselves binary, with one of these maps being transparent.

import numpy as np
import matplotlib.pyplot as plt
import s3dlib.surface as s3d
import s3dlib.cmap_utilities as cmu
#.. Klien Glass
# 1. Define function to examine .....................................
def klein(rtp) :
r,t,p = rtp
u = p
v = t
cU, sU = np.cos(u), np.sin(u)
cV, sV = np.cos(v), np.sin(v)
x = -(2/15)*cU* \
( ( 3 )*cV + \
( -30 + 90*np.power(cU,4) - 60*np.power(cU,6) + 5*cU*cV )*sU \
)
y = -(1/15)*sU* \
( ( 3 - 3*np.power(cU,2) -48*np.power(cU,4) +48*np.power(cU,6) )*cV + \
(-60 + ( 5*cU - 5*np.power(cU,3) - 80*np.power(cU,5) + 80*np.power(cU,7) )*cV )*sU \
)
z = (2/15)*( 3 + 5*cU*sU )*sV
return x,y,z
# 2. Setup and map surfaces .........................................
rez = 6
cmu.hue_cmap( 0.834,smooth=5.0, name='mycm')
cmu.alpha_cmap('mycm',0.0,name='blank')
cmu.stitch_cmap('mycm','blank',name='mycm_b')
glass = cmu.mirrored_cmap('mycm_b','glass', rev=True)
surface = s3d.SphericalSurface(rez,basetype='octa_c', linewidth=0 )
surface.map_geom_from_op( klein, returnxyz=True )
surface.transform(s3d.eulerRot(0,-90),translate=[0,0,2])
# 3. Construct figure, add surface, plot ............................
fig = plt.figure(figsize=plt.figaspect(1), facecolor='k')
ax = plt.axes(projection='3d', facecolor='k', aspect='equal')
ax.set_axis_off()
ax.view_init(20,-140)
s3d.auto_scale(ax,surface,uscale=.75)
surface.map_cmap_from_normals(glass,ax).fade(ax=ax)
ax.add_collection3d(surface.shade(ax=ax).hilite(focus=2) )
fig.tight_layout(pad=1)
plt.show()