Outer GlowΒΆ
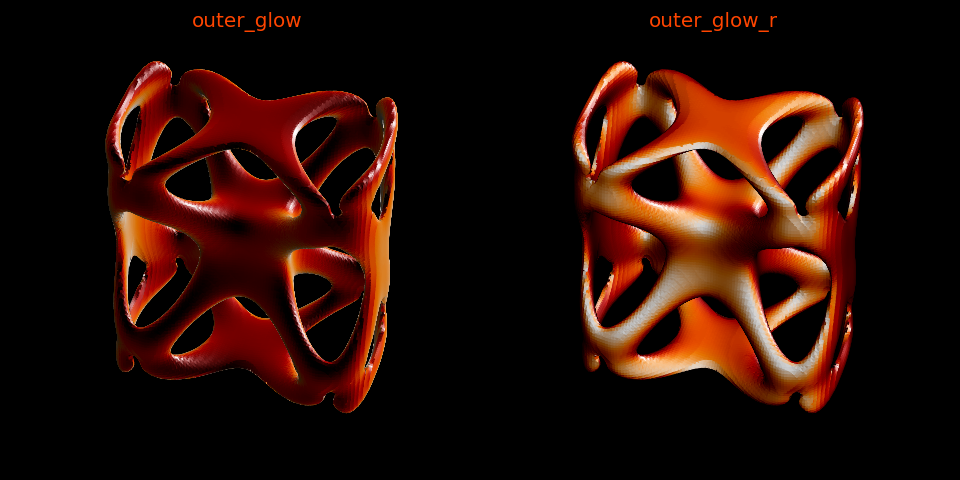
Outer background light is simulated using a mirrored colormap for mapping normal relative to the view.

The formula was found in an answer at math stackexchange .
import numpy as np
import matplotlib.pyplot as plt
import s3dlib.surface as s3d
import s3dlib.cmap_utilities as cmu
#.. Outer glow using mirrored colormaps.
def bc_surf(xyz) :
x,y,z = xyz
V,A = 0.85, 0.12
f1 = ((3*(x**2-1)*x**2) + 2*y**2)**2
f2 = ((z**2-V)**2)*((3*(y**2-1)*y**2) + 2*z**2)**2
f3 = ((x**2-V)**2)*((3*(z**2-1)*z**2) + 2*x**2)**2
f4 = ((y**2-V)**2)*(-A)
return f1+f2+f3+f4
mcop = cmu.mirrored_cmap('gist_heat','outer_glow')
mcop_r = cmu.mirrored_cmap('gist_heat','outer_glow_r', rev=True )
# ...................................................................
fig = plt.figure(figsize=plt.figaspect(.5), facecolor='k')
for i,cmap in enumerate([ mcop, mcop_r]) :
ax = fig.add_subplot(121+i, projection='3d', facecolor='k', aspect='equal')
ax.set_title(cmap.name, color='orangered', fontsize='x-large')
ax.set_axis_off()
ax.view_init(40,-75)
surface = s3d.Surface3DCollection.implsurf( bc_surf, 10, 1.1)
s3d.auto_scale(ax,surface,uscale=.8)
surface.map_cmap_from_normals(cmap,ax)
ax.add_collection3d(surface.shade(ax=ax).hilite(focus=2) )
fig.tight_layout(pad=2)
plt.show()