Inner GlowΒΆ
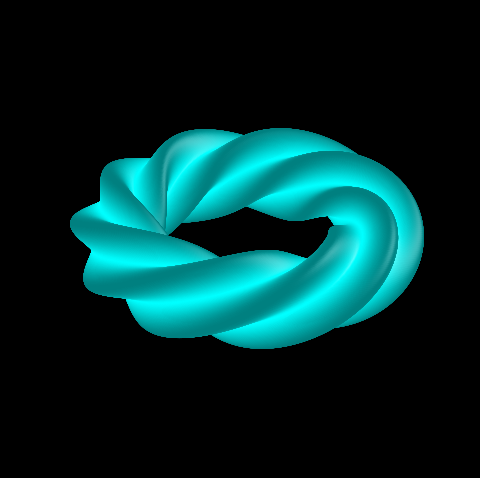
Internal light is simulated using a color gradient from the center of the torus and external highlighting, without shading.

import numpy as np
from matplotlib import pyplot as plt
import s3dlib.surface as s3d
import s3dlib.cmap_utilities as cmu
#.. simulation of an inner glow.
# 1. Define functions to examine ....................................
def twisted_star(rtz,twists) :
r,t,z = rtz
pts,mag,ratio = 4, 0.4,0.2
iRad = 1+mag*(1-np.cos(pts*(z+1)*np.pi) )
phi =t*twists
Z = ratio*iRad*np.sin(z*np.pi+phi)
R = r + ratio*iRad*np.cos(z*np.pi+phi)
return R,t,Z
def center_distance(rtz) :
r,t,z = rtz
d = np.array( [r,z] ) - np.tile([1,0.0],(len(r),1) ).T
return np.linalg.norm(d,axis=0)
# 2. Setup and map surfaces .........................................
rez=7
cmap = cmu.hsv_cmap_gradient([0.5,1,1],[0.5,1,0.5],'innerlite')
torus = s3d.CylindricalSurface(rez, basetype='squ', cmap=cmap)
torus.map_geom_from_op( lambda rtz : twisted_star(rtz,2) )
torus.map_cmap_from_op( center_distance )
# 3. Construct figure, add surfaces, and plot ......................
minmax=(-1.07,1.07)
fig = plt.figure(figsize=plt.figaspect(1))
ax = plt.axes(projection='3d',facecolor='k', aspect='equal')
ax.set(xlim=minmax, ylim=minmax, zlim=minmax )
ax.set_axis_off()
ax.add_collection3d(torus.hilite(0.35,focus=2))
fig.tight_layout(pad=0)
plt.show()