Base Class Surface 2ΒΆ
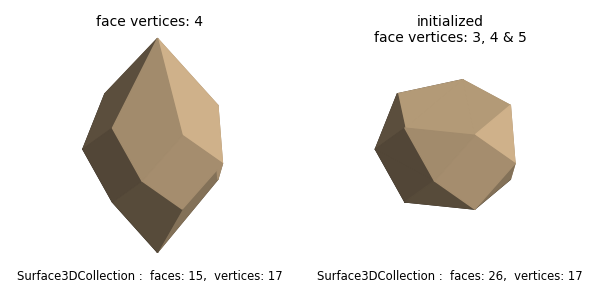
If the number of sides per face is not the same for the entire collection of surface faces, faces are further subdivided into triangles. In addition, the list of edges is not required to instantiate a base object, in which case, face edges are determined upon object creation.
For the example on the left, a list of 17 vertices is used to construct a surface of 15 quadrilateral faces.
For the example on the right, the same vertex list is used to construct a surface of 2 pentagon, 5 quadrilateral and 10 triangle faces for a total of 17 faces. For this surface object:
only 15 of the 17 vertices are used, however the constructed object will contain all 17 vertices.
the pentagon and quadrilateral faces are divided into 3 and 2 triangles, respectively. As a result, the surface object will have 26 faces, not 17. The subdivisions are illustrated by the plot of surface edges shown below.

It is suggested that for surfaces constructed from faces with varying number of sides, the edge list argument should be avoided since the edge list order and length will depend on the triangulation.
import numpy as np
from matplotlib import pyplot as plt
import s3dlib.surface as s3d
#.. Base Class Surfaces
# 1. Define function to examine ....................................
verts,R,H = [None]*17 , np.cos(np.pi/5),np.sin(np.pi/5)
for i in range(0,5) :
verts[i] = [np.cos(2*i*np.pi/5),np.sin(2*i*np.pi/5),0.0]
for i in range(5,10) :
verts[i] = [R*np.cos((1+2*i)*np.pi/5),R*np.sin((1+2*i)*np.pi/5), H]
verts[i+5] = [R*np.cos((1+2*i)*np.pi/5),R*np.sin((1+2*i)*np.pi/5),-H]
verts[15] = [0.0,0.0, 1/H]
verts[16] = [0.0,0.0,-1/H]
faceIndices_4 = [
[15, 5, 1, 6], [15, 6, 2, 7], [15, 7, 3, 8], [15, 8, 4, 9], [15, 9, 0, 5],
[ 5, 0,10, 1], [ 6, 1,11, 2], [ 7, 2,12, 3], [ 8, 3,13, 4], [ 9, 4,14, 0],
[16,11, 1,10], [16,12, 2,11], [16,13, 3,12], [16,14, 4,13], [16,10, 0,14]
]
faceIndices_345 = [
[ 5, 6, 7, 8, 9 ],
[0, 5, 9], [1, 6, 5], [2, 7, 6], [3, 8, 7], [4, 9, 8],
[5, 0,10, 1], [6, 1,11, 2], [7, 2,12, 3], [8, 3,13, 4], [9, 4,14, 0],
[0,14,10], [1,10,11], [2,11,12], [3,12,13], [4,13,14],
[ 14,13,12,11,10 ]
]
# 2. Setup and map surface .........................................
surface_4 = s3d.Surface3DCollection(verts,faceIndices_4, color='tan')
surface_345 = s3d.Surface3DCollection(verts,faceIndices_345,color='tan')
surfaces = [surface_4, surface_345]
# 3. Construct figure, add surface, and plot ......................
minmax = (-1.0,1.0)
fig = plt.figure(figsize=(6,3))
fig.text(0.25,0.95,"face vertices: 4", ha='center', va='top')
fig.text(0.75,0.95,"initialized\nface vertices: 3, 4 & 5", ha='center', va='top')
for i in range(len(surfaces)) :
fig.text(0.25+0.5*i,0.05,str(surfaces[i]), ha='center', va='bottom',fontsize='small')
ax =fig.add_subplot(121+i, projection='3d', aspect='equal')
ax.set(xlim=minmax, ylim=minmax, zlim=minmax)
ax.set_axis_off()
ax.set_proj_type('ortho')
ax.add_collection3d(surfaces[i].shade())
#ax.add_collection3d(surfaces[i].edges.fade(.05))
fig.tight_layout(pad=1)
plt.show()