Clipped SurfaceΒΆ
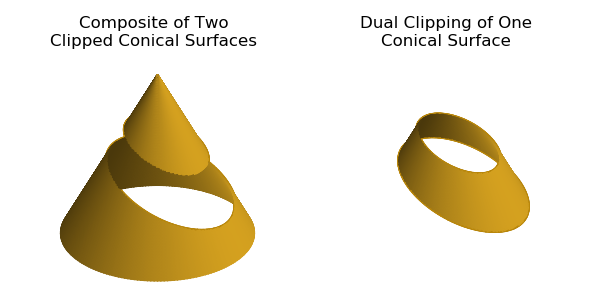
A discussion of surface clipping is found in the Contours, Clipped Surfaces and Line Projection and Slices guide.
import matplotlib.pyplot as plt
import s3dlib.surface as s3d
import numpy as np
import copy
#.. Conical Surface Clipping
# 1. Define function to examine ....................................
def cone_cylnd(rtz) :
r,t,z = rtz
R = 0.5*(1.00001-z)
return R,t,z
# 2. Setup and map surface .........................................
rez=6
dir_up,dir_dn,posDist = [0.5,0,1],[-.5,0,-1], 0.2
negDist = -posDist
cone = s3d.CylindricalSurface(rez).map_geom_from_op(cone_cylnd)
cone.set_color('goldenrod')
cone.shade()
bot_cone = copy.copy(cone)
top_cone = copy.copy(cone)
mid_cone = copy.copy(cone)
bot_cone.clip_plane(negDist, direction=dir_up)
top_cone.clip_plane(negDist, direction=dir_dn)
com_cone = (top_cone + bot_cone)
mid_cone.clip_plane(posDist, direction=dir_up)
mid_cone.clip_plane(posDist, direction=dir_dn)
top_ellipse = cone.contourLines(posDist, direction=dir_up, coor='p')
bot_ellipse = cone.contourLines(negDist, direction=dir_up, coor='p')
edge_line = (bot_ellipse + top_ellipse)
edge_line.set_linewidth(3)
edge_line.set_color('darkgoldenrod')
edge_line2 = copy.copy(edge_line)
# 3. Construct figure, add surface, and plot ......................
minmax = (-0.8,0.8)
fig = plt.figure(figsize=(6,3))
ax1 = fig.add_subplot(121, projection='3d', aspect='equal')
ax1.set(xlim=minmax, ylim=minmax, zlim=minmax)
ax1.set_title('Composite of Two\nClipped Conical Surfaces', pad=-1)
ax1.set_axis_off()
ax1.add_collection3d(com_cone)
ax1.add_collection3d(edge_line)
ax2 = fig.add_subplot(122, projection='3d', aspect='equal')
ax2.set(xlim=minmax, ylim=minmax, zlim=minmax)
ax2.set_title('Dual Clipping of One\nConical Surface', pad=-1)
ax2.set_axis_off()
ax2.add_collection3d(mid_cone)
ax2.add_collection3d(edge_line2)
fig.tight_layout()
plt.show()