Vector Field Domain FacesΒΆ
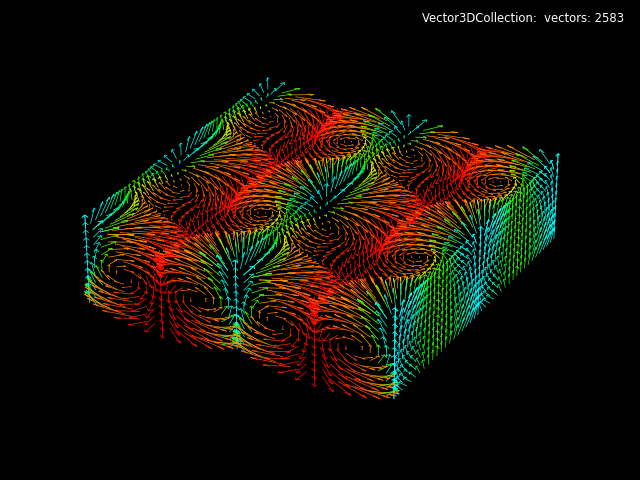
The following custom colormap was constructed to emphasize the positive vertical component of the vector direction.

import numpy as np
from matplotlib import pyplot as plt
import s3dlib.surface as s3d
import s3dlib.cmap_utilities as cmu
#.. Vector Field Visualization
# 1. Define function to examine ....................................
def vfield(xyz) :
x,y,z = xyz
mult,f,g,h = 0.075, 2, 0.1, 1
u = np.sin(2.0*np.pi*x) * np.sin(0.5*np.pi*z)
v = np.sin(2.0*np.pi*y) * np.sin(0.5*np.pi*z)
w = 4.0*np.cos(2.0*np.pi*x)
u = (1+f*np.cos(2*np.pi*y))*u
w = (1+g*np.cos(2*np.pi*y))*w
v = (1+h*np.cos(2*np.pi*y))*v
return mult*np.array([u,v,w])
# Make the grid sides...
x,y = np.meshgrid(np.arange(-1, 1.05, 0.05), np.arange(-1, 1.05, 0.05) )
z = np.ones_like(x)
xy_plane = np.reshape(np.array( [x,y,z] ).T,(-1,3))
x,z = np.meshgrid(np.arange(-1, 1.05, 0.05), np.arange(-1, 1.20, 0.2) )
y = -np.ones_like(x)
xz_plane = np.reshape(np.array( [x,y,z] ).T,(-1,3))
y,z = np.meshgrid(np.arange(-1, 1.05, 0.05), np.arange(-1, 1.20, 0.2) )
x = np.ones_like(y)
yz_plane = np.reshape(np.array( [x,y,z] ).T,(-1,3))
location = np.concatenate( (xy_plane,xz_plane,yz_plane),axis=0 )
vect = vfield(location.T).T
# 2. Setup and map vectors ........................................
bot = cmu.hue_cmap(0.0,0.1)
top = cmu.hue_cmap(0.1,0.5)
cmap = cmu.stitch_cmap(bot,top,bndry=0.8,name='Z_dot')
VF = s3d.Vector3DCollection(location,vect,lw=0.5,alr=0.2)
VF.map_cmap_from_direction( cmap,[0,0,1] )
# 3. Construct figure, add surface, and plot ......................
f = 0.8
fig = plt.figure(facecolor='k')
fig.text(0.975,0.975,str(VF),color='w',
ha='right', va='top', fontsize='smaller', multialignment='right')
ax = plt.axes(projection='3d',facecolor='k', aspect='equal')
ax.set(xlim=(-f,f), ylim=(-f,f), zlim=(-4*f,4*f) )
ax.set_axis_off()
ax.add_collection3d(VF)
fig.tight_layout(pad=0)
plt.show()