Constant Z Contours of a Function.ΒΆ
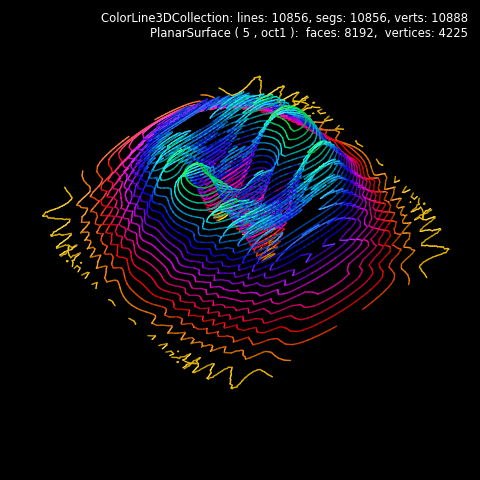
For this case, contour line colors are taken from the colored source surface instead of color mapping the lines. As a result, the shading of the contour lines are enhanced using the surface shading.
import numpy as np
import matplotlib.pyplot as plt
import s3dlib.surface as s3d
import s3dlib.cmap_utilities as cmu
#.. PlanarSurface contour line plot
# 1. Define function to examine .....................................
def fun011(xyz) :
x,y,z = xyz
X2, Y2 = x**2, y**2
a,b = 0.04, 0.06
R2 = a*X2 + b*Y2
one = np.ones(len(x))
f = np.sin( X2 + 0.1*Y2)/( 0.1*one + R2 )
g = ( X2 + 1.9*Y2) * np.exp( one - R2)/4
Z = f + g
return x,y,Z
# 2. Setup and map surfaces .........................................
rez=5
cmap = cmu.hsv_cmap_gradient([1.166,1,1],[0.333,1,1])
surface = s3d.PlanarSurface(rez,basetype='oct1',linewidth=.3)
surface.transform(scale=10)
surface.map_geom_from_op(fun011)
surface.map_cmap_from_op( cmap=cmap )
surface.shade(.5).hilite(.3)
line= surface.contourLineSet(20,direction=[0,0,1])
line.set_linewidth(1)
# 3. Construct figures, add surface, plot ...........................
fig = plt.figure(figsize=plt.figaspect(1), facecolor='black')
fig.text(0.975,0.975,str(line)+'\n'+str(surface), ha='right', va='top',
fontsize='smaller', multialignment='right', color='white')
ax = plt.axes(projection='3d', facecolor='black', aspect='equal')
minmax = (-7.5,7.5)
ax.set(xlim=minmax, ylim=minmax, zlim=minmax)
ax.set_axis_off()
ax.set_proj_type('ortho')
ax.view_init(50,140)
ax.add_collection3d(line)
fig.tight_layout(pad=2)
plt.show()