ContoursΒΆ
This is a 3D comparison to the 2D contours Matplotlib examples. A larger number of vertices were used in this particular example, compared to 256, to provide a smooth 3D surface.
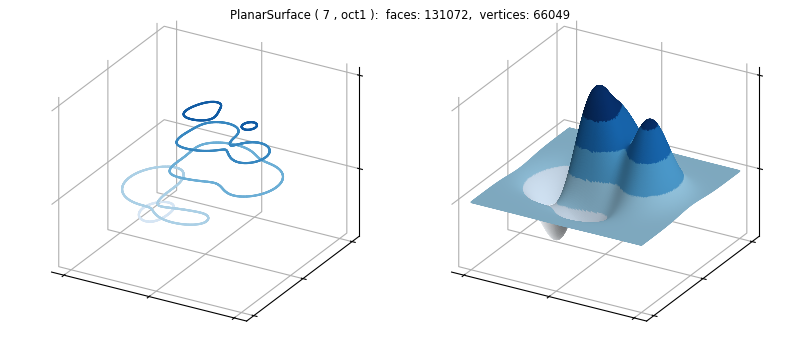
import numpy as np
import matplotlib.pyplot as plt
from matplotlib import colormaps,colors
import s3dlib.surface as s3d
#.. Matplotllib Examples: contour(x, y, z) and contourf(x, y)
def z_func(xyz) :
x,y,z = xyz
Z = (1 - x/2 + x**5 + y**3) * np.exp(-x**2 - y**2)
return x,y,Z
# 2. Setup and map surface .........................................
sixColors = colormaps['Blues'](np.linspace(0, 1, 6))
sixColor_cmap = colors.ListedColormap(sixColors)
rez = 7
surface = s3d.PlanarSurface(rez,'oct1',cmap="Blues").domain(3,3)
surface.map_geom_from_op(z_func)
surface.map_cmap_from_op() # default: z-direction
contours = surface.contourLineSet(5) # default: planar, z-direction
surface.map_cmap_from_op( cmap=sixColor_cmap)
# 3. Construct figure, add surface, and plot ......................
fig = plt.figure(figsize=(8,3.5))
fig.text(0.5,0.975,str(surface), ha='center', va='top', fontsize='smaller')
for i in range(2) :
ax = fig.add_subplot(121+i, projection='3d')
ax.set(xticks=[-3,0,3],yticks=[-3,0,3],zticks=[0,1])
ax.tick_params(labelcolor='w')
ax.set_proj_type('ortho')
ax.xaxis.set_pane_color([1,1,1])
ax.yaxis.set_pane_color([1,1,1])
ax.zaxis.set_pane_color([1,1,1])
s3d.auto_scale(ax,surface)
if True : # <-- show 3D view..........
ax.add_collection3d(contours.fade(.2) if i==0 else surface.shade())
else : # <-- show x-y plane........
ax.view_init(90,-90)
ax.add_collection3d(contours if i==0 else surface)
fig.tight_layout(pad=0)
plt.show()
The 2D plot can be visualized by rotating the plot using ax.view_init(90,-90) and omitting any surface shading.
