(x,y) Dataset Density¶
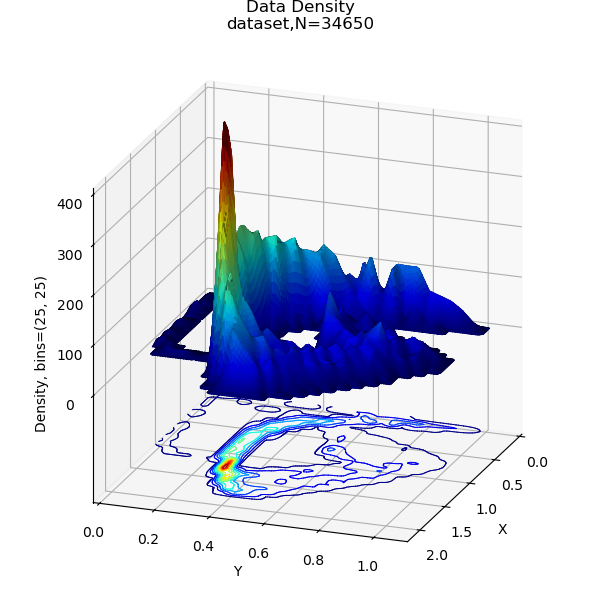
This example demonstrates a 3D data density visualization. A detailed dataset was generated based on the swirl surface face characteristics, as shown in the Surface Face Distributions example.
The 2D density plots using the Matplotlib axis functions are shown below.
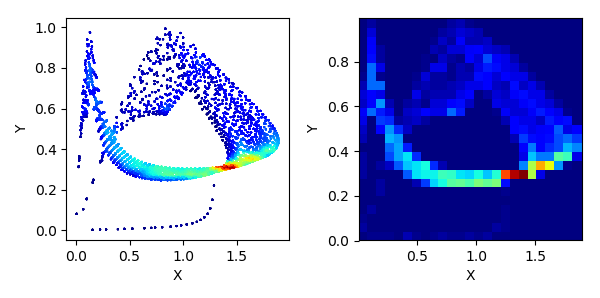
import numpy as np
import matplotlib.pyplot as plt
import s3dlib.surface as s3d
from matplotlib import cm,colormaps
#.. Data density figures .....
# generate a x,y dataset ..............................................
bns = (25,25)
surface = s3d.SphericalSurface.grid(5*20,7*25)
surface.map_geom_from_op(lambda c: [2+np.sin(7*c[1]+5*c[2]),c[1],c[2]] )
data = np.array(surface.area_h2b) # ................... entire dataset [x,y]
scale = 1
f = s3d.density_function(data,bins=bns, scale=scale) # returns density = f(x,y)
# FIGURE 1 : Distribution Surface ===============================================
# 1. Define function to examine .......................................
def surfDist(xyz):
x,y,z=xyz
return x,y,f(x,y) # use density function for Z
# 2. Setup and map surfaces .........................................
rez,cmap = 7, colormaps['jet']
surface = s3d.PlanarSurface(rez,cmap=cmap).domain([0,2.2],[0,1.2])
surface.map_geom_from_op(surfDist)
surface.map_cmap_from_op(lambda c: c[2])
contours = surface.contourLineSet(10)
contours = contours + surface.contourLines(5.0) #.. add lower contour
contours.set_linewidth(0.75)
contours.map_to_plane(-200)
surface.clip( lambda c: c[2] > 0.01 )
# 3. Construct figure, add surface, plot ............................
xticks = [0.0,0.5,1.0,1.5,2.0]
yticks = [0.0,0.2,0.4,0.6,0.8,1.0]
zticks = [0,100,200,300,400]
fig = plt.figure(figsize=(6,6))
ax = plt.axes(projection='3d', aspect='equal')
title = "Data Density\ndataset,N="+ str(len(data[0]))
ax.set_title( title )
ax.set_proj_type('ortho')
zlabel = 'Density, bins='+str(bns)
ax.set(xlabel='X',ylabel='Y',zlabel=zlabel,
xlim=[0,2.2],ylim=[0,1.1],zlim=[-200,400],
xticks=xticks,yticks=yticks,zticks=zticks)
ax.view_init(20,20)
ax.add_collection3d(contours)
ax.add_collection3d(surface.shade())
fig.tight_layout(pad=0)
# FIGURE 2 : Data plot & 2D histogram ===========================================
# 3. Construct figure, 2D plots ............................
fvals = f(*data)/np.amax(f(*data)) # .......... normalize to [0,1]
colors = [ cmap(z) for z in fvals ] # .......... data colors
fig = plt.figure(figsize=(6,3))
ax = fig.add_subplot(121)
ax.set( xlabel='X',ylabel='Y',xticks=xticks,yticks=yticks)
ax.scatter(*data,s=1,marker='.',c=colors)
ax = fig.add_subplot(122)
ax.set( xlabel='X',ylabel='Y',xticks=xticks,yticks=yticks)
ax.hist2d(*data,bins=bns, cmap=cmap)
fig.tight_layout()
# ==============================================================================
plt.show()