Edge to Edge Surface 2ΒΆ
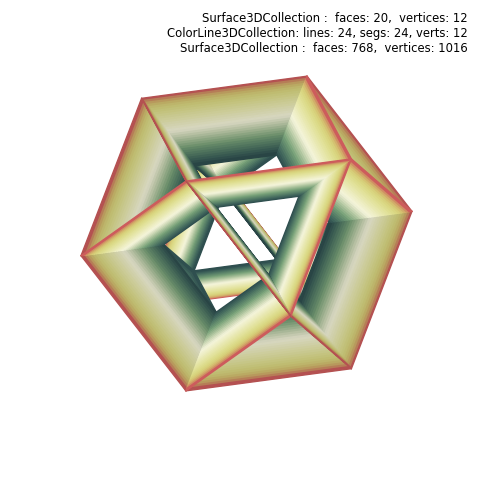
Surface vertex and face indices are defined in a similar manner as the Base Class Geometric Mapping example. The construction is similar to that used for the Edge to Edge Surface example with the minor exception of:
the line is assigned the initedges surface property (highlighted) instead of the edge property since initial faces are assigned both triangular and quadrilateral faces. The actual surface faces are all triangular so the surface edges were not used.
to emphasize the Truncated Cube edges, a custom colormap is used, as shown below:

import copy
import numpy as np
import matplotlib.pyplot as plt
import s3dlib.surface as s3d
import s3dlib.cmap_utilities as cmu
#.. Truncated Cube Edges to Edges Surface
# 1. Define Truncated Cube geometry ...........................
v = [
[ 1, 0, 1], [ 0, 1, 1], [-1, 0, 1], [ 0,-1, 1],
[ 1, 1, 0], [-1, 1, 0], [-1,-1, 0], [ 1,-1, 0],
[ 1, 0,-1] ,[ 0, 1,-1], [-1, 0,-1], [ 0,-1,-1] ]
f3 = [
[ 0, 4, 1], [ 1, 5, 2], [ 2, 6, 3], [ 3, 7, 0],
[ 9, 4, 8], [10, 5, 9], [11, 6,10], [ 8, 7,11] ]
f4 = [
[ 0, 1, 2, 3], [ 8,11,10, 9],
[ 0, 7, 8, 4], [ 1, 4, 9, 5], [ 2, 5,10, 6], [3, 6,11, 7] ]
f = f3 + f4
# 2. Setup and map surface .........................................
cmA = cmu.hsv_cmap_gradient('darkslategrey','beige',smooth=1)
cmB = cmu.hsv_cmap_gradient('beige','indianred',smooth=8)
cmu.stitch_cmap(cmA,cmB,name='rhom')
lrez = 5
surface = s3d.Surface3DCollection(v, f, name='Truncated Cube')
edge = surface.initedges
outerEdge = copy.copy(edge).transform(scale=2.0)
fsurf = outerEdge.get_surface_to_line(edge,lrez=lrez)
fsurf.map_cmap_from_op( lambda c : s3d.SphericalSurface.coor_convert(c)[0],'rhom')
# 3. Construct figure, add surface plot ............................
fig = plt.figure(figsize=plt.figaspect(1))
fig.text(0.975,0.975, str(surface)+'\n'+str(edge)+'\n'+str(fsurf),
ha='right', va='top', fontsize='smaller', multialignment='right')
ax = plt.axes(projection='3d', aspect='equal')
w = 0.8*fsurf.bounds['rorg'][1]
minmax = (-w,w)
ax.set(xlim=minmax, ylim=minmax, zlim=minmax)
ax.set_axis_off()
ax.set_proj_type('ortho')
ax.add_collection3d(fsurf.shade(0.85,isAbs=True))
fig.tight_layout(pad=0)
plt.show()